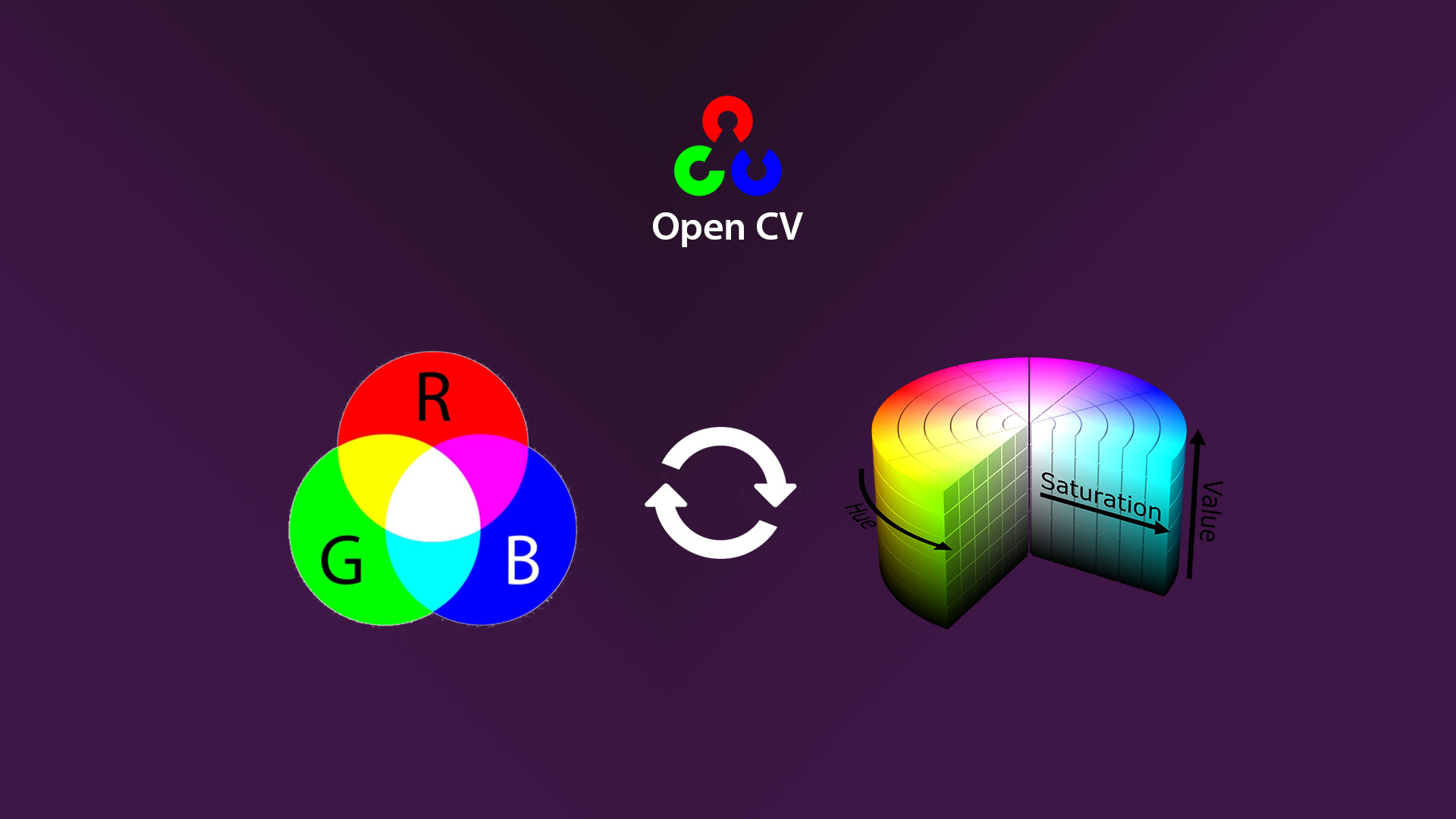
Hello Folks ! Today I will be sharing a solution of one of the most common problem that we face while working on image processing i.e. getting values of HSV from RGB colorspace. Usually we encounter this problem when we need to segment out specific part of image using OpenCv’s classis inRange
function. While using this function we need to provide color range of desired area of image in HSV format as color processing is far more convenient in HSV colorspace.
Though we have lots of solutions online for this issue but most of them do not provide values compatible with OpenCV, so I decided to make my own interactive program for this purpose.
Before starting with the program itself lets first understand what HSV color model is.
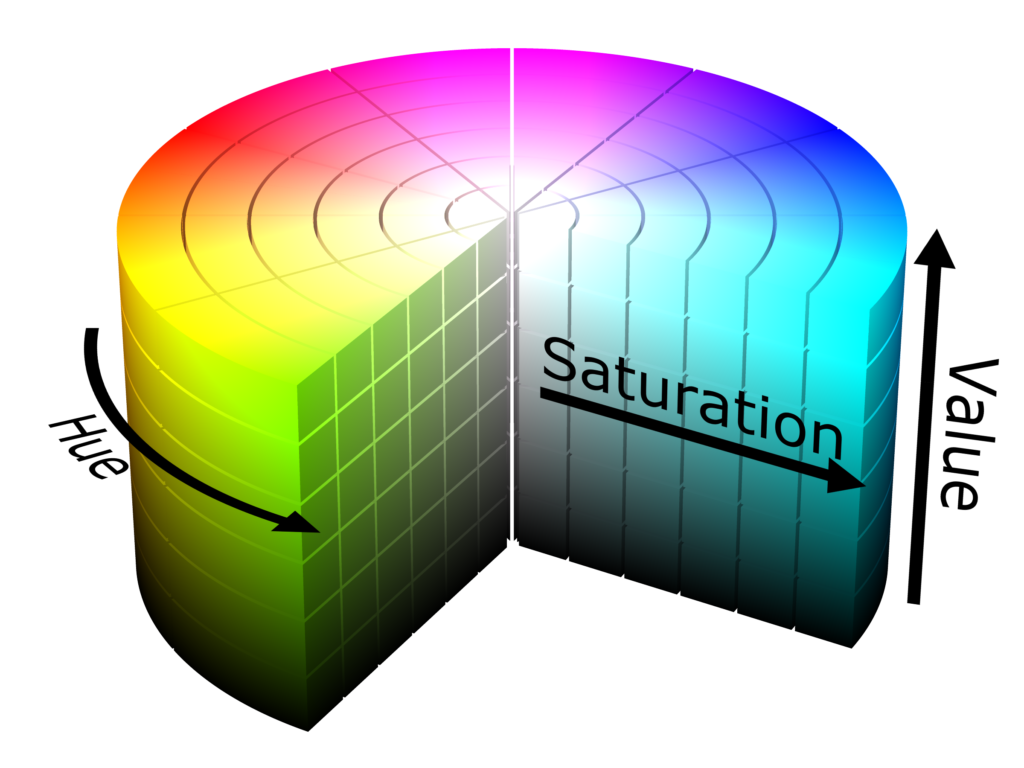
The HSV color wheel sometimes appears as a cone or cylinder, but always with these three components:
HUE
Hue is the color portion of the model, expressed as a number from 0 to 360 degrees:
- Red falls between 0 and 60 degrees.
- Yellow falls between 61 and 120 degrees.
- Green falls between 121 and 180 degrees.
- Cyan falls between 181 and 240 degrees.
- Blue falls between 241 and 300 degrees.
- Magenta falls between 301 and 360 degrees.
SATURATION
Saturation describes the amount of gray in a particular color, from 0 to 100 percent. Reducing this component toward zero introduces more gray and produces a faded effect. Sometimes, saturation appears as a range from 0 to 1, where 0 is gray, and 1 is a primary color.
VALUE (OR BRIGHTNESS)
Value works in conjunction with saturation and describes the brightness or intensity of the color, from 0 to 100 percent, where 0 is completely black, and 100 is the brightest and reveals the most color.
Coding Time
import cv2 import numpy as np import sys def rgb_hsv(): n = str(input("Enter RGB values seperated by comma : ")) try: r,g,b = n.split(",") h,s,v = cv2.cvtColor(np.uint8([[[b,g,r]]]),cv2.COLOR_BGR2HSV)[0][0] print(f"\nResult : H = {h}, S = {s}, V = {v}") except: print("\nInvalid input format\n") def hsv_rgb(): n = str(input("Enter HSV values seperated by comma : ")) try: h,s,v = n.split(",") r,g,b = cv2.cvtColor(np.uint8([[[h,s,v]]]),cv2.COLOR_HSV2RGB)[0][0] print(f"\nResult : R = {r}, G = {g}, B = {b}") except: print("\nInvalid input format\n") def main(): while True: cstr = " Program For Color Space Conversion " print("\n"*2+cstr.center(100, '*')+"\n") try: i = int(input("Select your option :\n(1) RGB TO HSV\n(2) HSV TO RGB\n(3) Exit\n")) if i == 1: rgb_hsv() elif i == 2: hsv_rgb() elif i == 3: sys.exit(1) else: print("\nWrong option selected\n") except SystemExit: sys.exit(1) except: print("\nInvalid input format\n") if __name__ == "__main__": main()
Explaination
Here I have written a interactive python shell program which will take RGB/HSV values from user and convert it to HSV/RGB format. I have made use OpenCV’s function cvtColor
for this purpose. The program is also smart enough to detect invalid user inputs and act accordingly.
For any queries or issues related to this post please comment below and I will be glad to help you 🙂 .