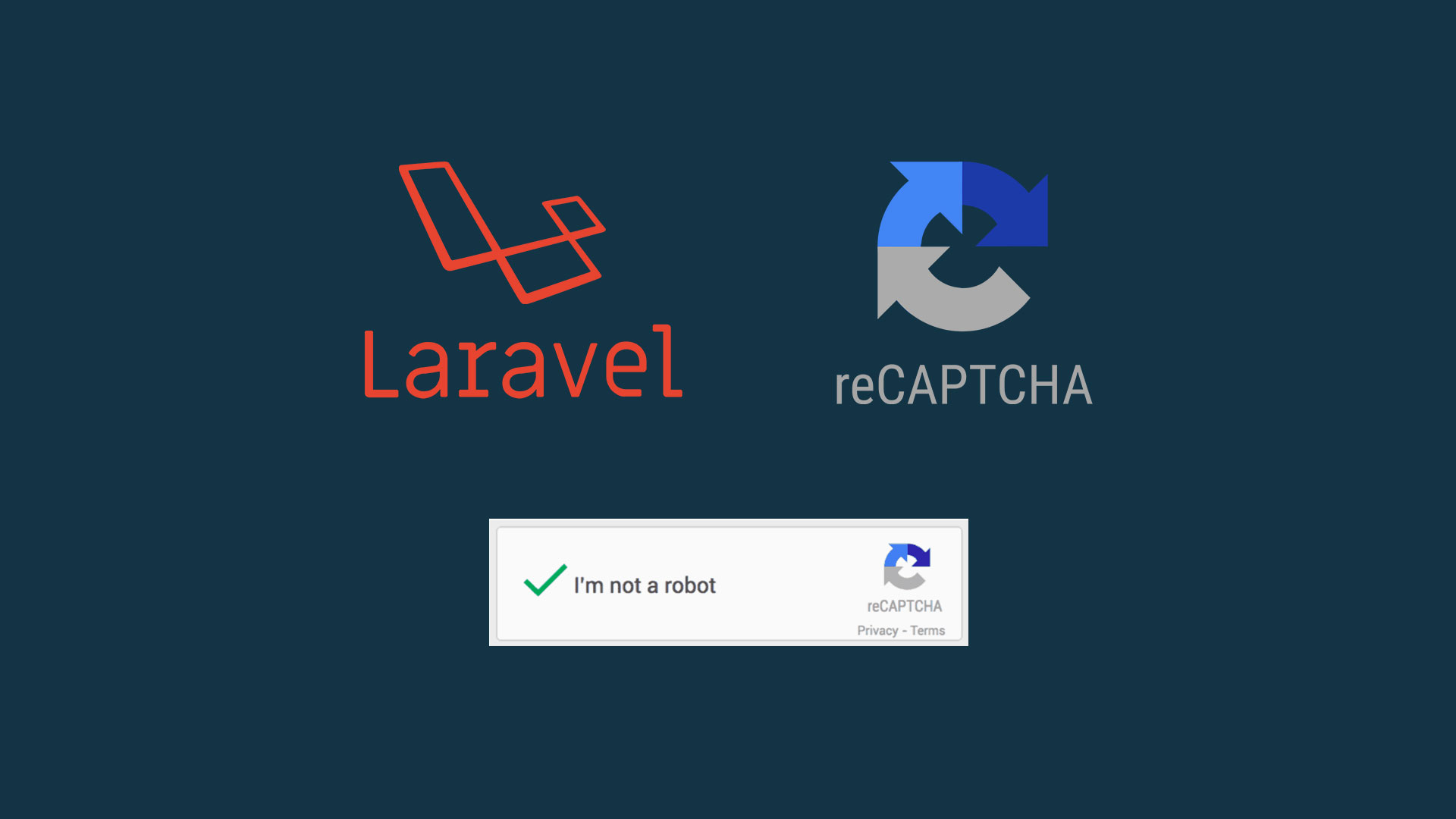
Hello Guys ! Today I will be showing you how to integrate Google reCaptcha with Laravel in 3 quick and easy steps. For those who are unaware about reCaptcha, below is the definition of it.
reCAPTCHA is a free service from Google that helps protect websites from spam and abuse. A “CAPTCHA” is a turing test to tell human and bots apart. It is easy for humans to solve, but hard for “bots” and other malicious software to figure out. By adding reCAPTCHA to a site, you can block automated software while helping your welcome users to enter with ease.
Also you can learn more about its uses you can go through its documentation here. Now proceeding further, in order to use the reCaptcha service of Google you need to create a reCaptcha account. The prcoess is very straightforward and easy to follow. Once the account is generated you will be provided with two keys namely GOOGLE_CAPTCHA_PUBLIC_KEY and GOOGLE_CAPTCHA_PRIVATE_KEY.
Once you have reCaptcha keys in your pocket you are good to proceed further and follow the below steps to successfully integrate reCaptcha into your website form 🙂 .
STEP 1 – Add reCaptcha Package
Navigate through your laravel project root directory and type below command :
composer require google/recaptcha
This will download the required package and add the dependency to the composer.json file.
STEP 2 – Create Captcha Rule
Now that we have our reCaptcha package installed in our project we can now use it to create a custom rule for our captcha.
Create a custom rule using the below command :
php artisan make:rule Captcha
Now open the generated rule file from app\Rules folder and add below code :
<?php namespace App\Rules; use Illuminate\Contracts\Validation\Rule; use ReCaptcha\ReCaptcha; class Captcha implements Rule { /** * Create a new rule instance. * * @return void */ public function __construct() { // } /** * Determine if the validation rule passes. * * @param string $attribute * @param mixed $value * @return bool */ public function passes($attribute, $value) { $captcha = new ReCaptcha('ENTER YOUR PRIVATE KEY'); $response = $captcha->verify($value, $_SERVER['REMOTE_ADDR']); return $response->isSuccess(); } /** * Get the validation error message. * * @return string */ public function message() { return 'Complete the reCAPTCHA to submit the form'; } }
With that in place our Step 2 is completed successfully.
STEP 3 – Implement reCaptcha in the Form
In this step 3 we will be integrating the Google reCaptcha field in our web form and add the custom rule (created in Step 2) in our form validation. So lets proceed .
Add reCaptcha Field
Open your web form template file and add below code inside your form tag :
<div class="g-recaptcha" data-sitekey="ENTER YOUR PUBLIC KEY"></div>
Also add the below js file under the script tags.
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
Add Validation In Controller
Now we need to check the validity of the input field added above.For this we will be using our custom rule created in Step 2. Add below code in your respective controller and don’t forget to import the Captcha rule using use App\Rules\Captcha
before using it.
public function form_response(Request $request) { $this->validate($request,[ 'g-recaptcha-response' => new Captcha() ]); // Code for processing the form }
That’s it ! We are done with the process (Umm…were you expecting some more ? 😉 ) . For any queries related to this tutorial please comment below and if you like my contents please make it social.
Hello, I think that I saw you visited my weblog, so I came to return the favor. I’m trying to find things to improve my web site! I suppose it’s okay to use some of your ideas!!